ESP32 with Soil Moisture Sensor on Web Server (MicroPython)
Monitor Soil Moisture with ESP32 board and Soil Moisture Sensor Module with Micro Python. Show Status on ESP32 Web Server.
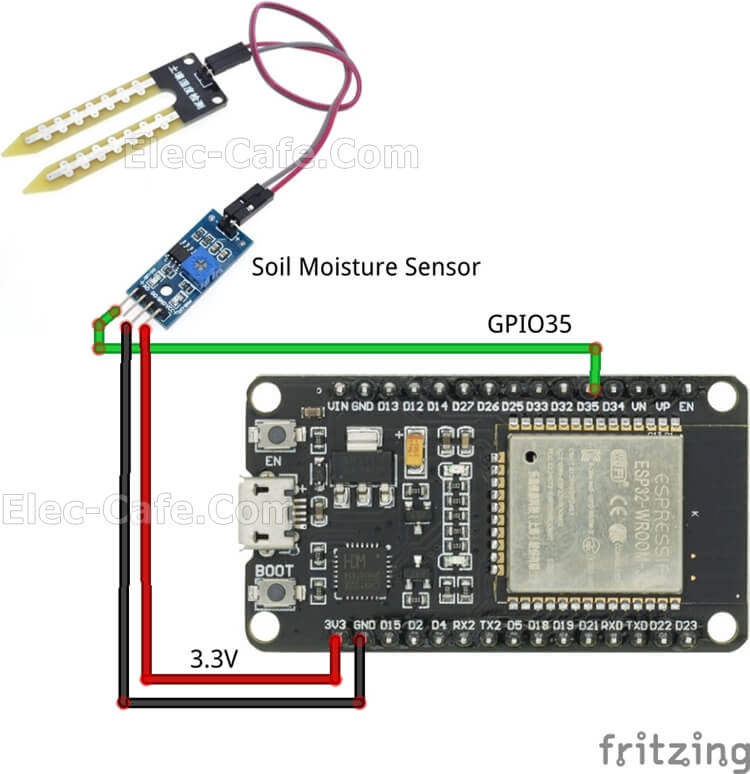
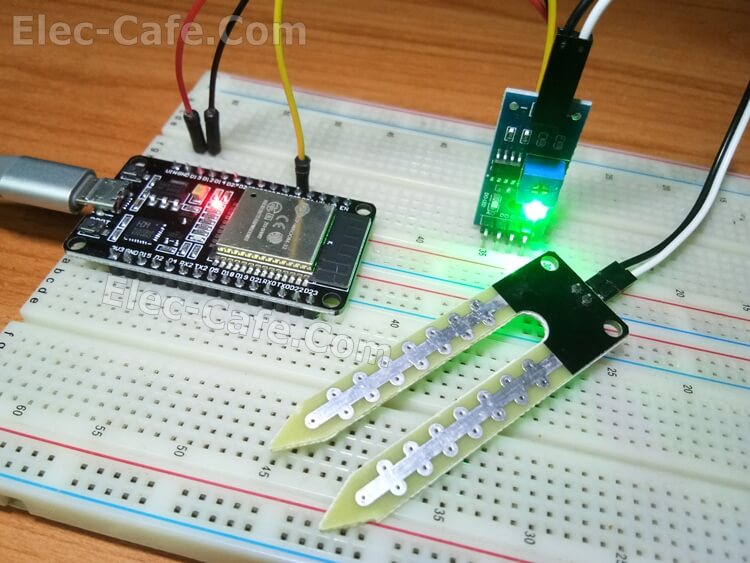
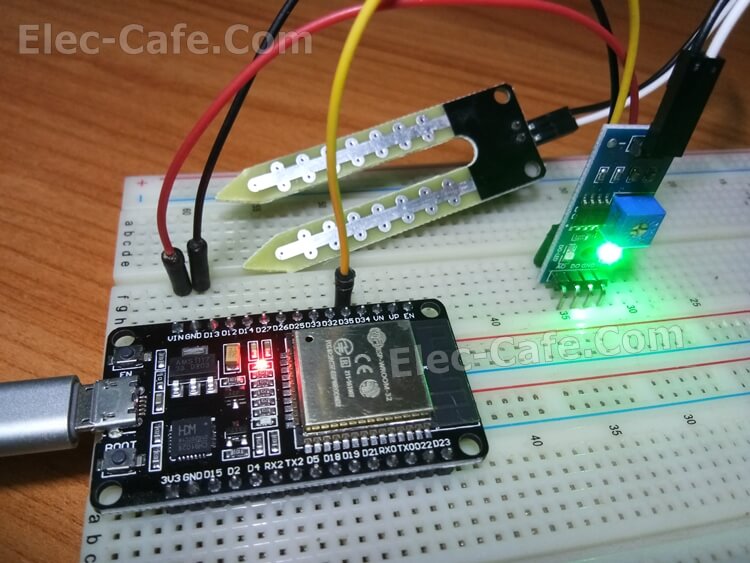
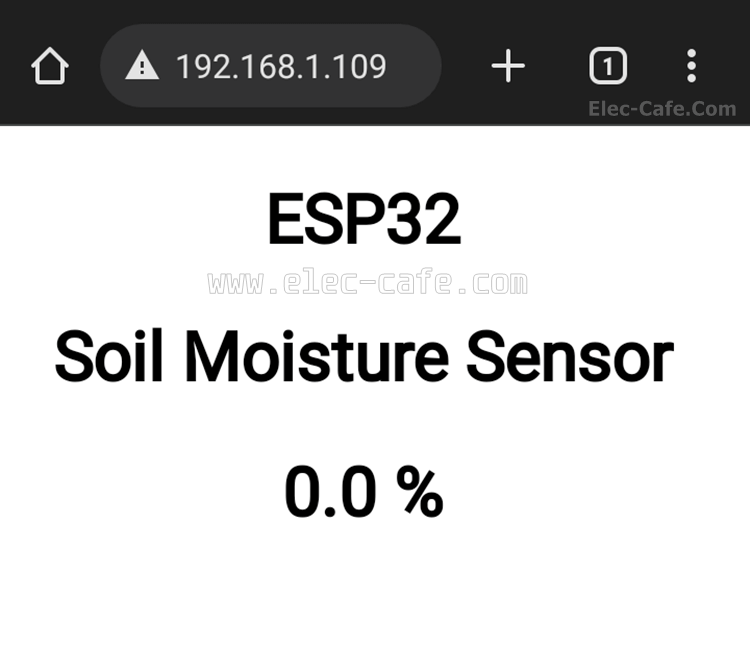
MicroPython Code – ESP32 with Soil Moisture Sensor on Web Server
from machine import Pin, SoftI2C
from machine import Pin, ADC
import network
import time
import socket
import _thread
# Soil Moisture
soil = ADC(Pin(35))
m = 100
min_moisture=0
max_moisture=4095
soil.atten(ADC.ATTN_11DB) #Full range: 3.3v
soil.width(ADC.WIDTH_12BIT) #range 0 to 4095
def check_moisture():
print('Check Moisture Starting...')
global m
while True:
try:
soil.read()
time.sleep(2)
m = (max_moisture-soil.read())*100/(max_moisture-min_moisture)
moisture = 'Soil Moisture: {:.1f} %'.format(m)
print("Soil Moisture: " + "%.1f" % m +"% (adc: "+str(soil.read())+")")
time.sleep(5)
except:
pass
# START
print('Starting...')
# WIFI
wifi = 'Your_ssid' # your wifi ssid
password = 'Your_Password' # your wifi password
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
time.sleep(3)
wlan.connect(wifi, password)
time.sleep(5)
status = wlan.isconnected() # True/False
ip ,_ ,_ ,_ = wlan.ifconfig()
if status == True:
print('IP:{}'.format(ip))
time.sleep(2)
print('Connected')
else:
print('Disconnected')
# HTML
html1 = '''
<!doctype html>
<html lang="en">
<body style="font-family:verdana;">
<div class="container">
<form>
<center>
<h2><b>ESP32<b></h2>
<h2><b>Soil Moisture Sensor<b></h2>
<h2>
'''
html2 = '''
</h2><br>
</center>
</form>
</div>
</body>
</html>
'''
# RUN
def runserver():
global m
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
host = ''
port = 80
s.bind((host,port))
s.listen(5)
while True:
client, addr = s.accept()
print('Connection from: ', addr)
data = client.recv(1024).decode('utf-8')
print([data])
moisture = '{:.1f} %'.format(m)
client.send(html1 + moisture + html2)
client.close()
_thread.start_new_thread(runserver,())
_thread.start_new_thread(check_moisture,())